- Published on
- · 4 min read
Building REST API using Spring boot
- Authors
- Name
- Nguyễn Tạ Minh Trung
Table of Contents
Introduction
Developers are no longer unfamiliar with the Restful API. Using the HTTP protocol, we may effortlessly communicate with other applications by using Restful API.... So, let's start a new Rest API with Spring boot.
Wait.... Before starting, we have to setup environments as a Java backend developers 👨🏻💻:
- Java - SDK Manager is recommended
- Maven or Gradle
- Code IDE - IntelliJ is recommended
Okay! The few things above are enough to get start... Let's go 🚀
Getting started
- In this article, I am going to using Maven for dependencies management, postgresql as database.
- Going to Spring Initializr and generating a new Spring project with following the dependencies:
- Spring Web
- Lombok
- postgresql
- Spring JPA
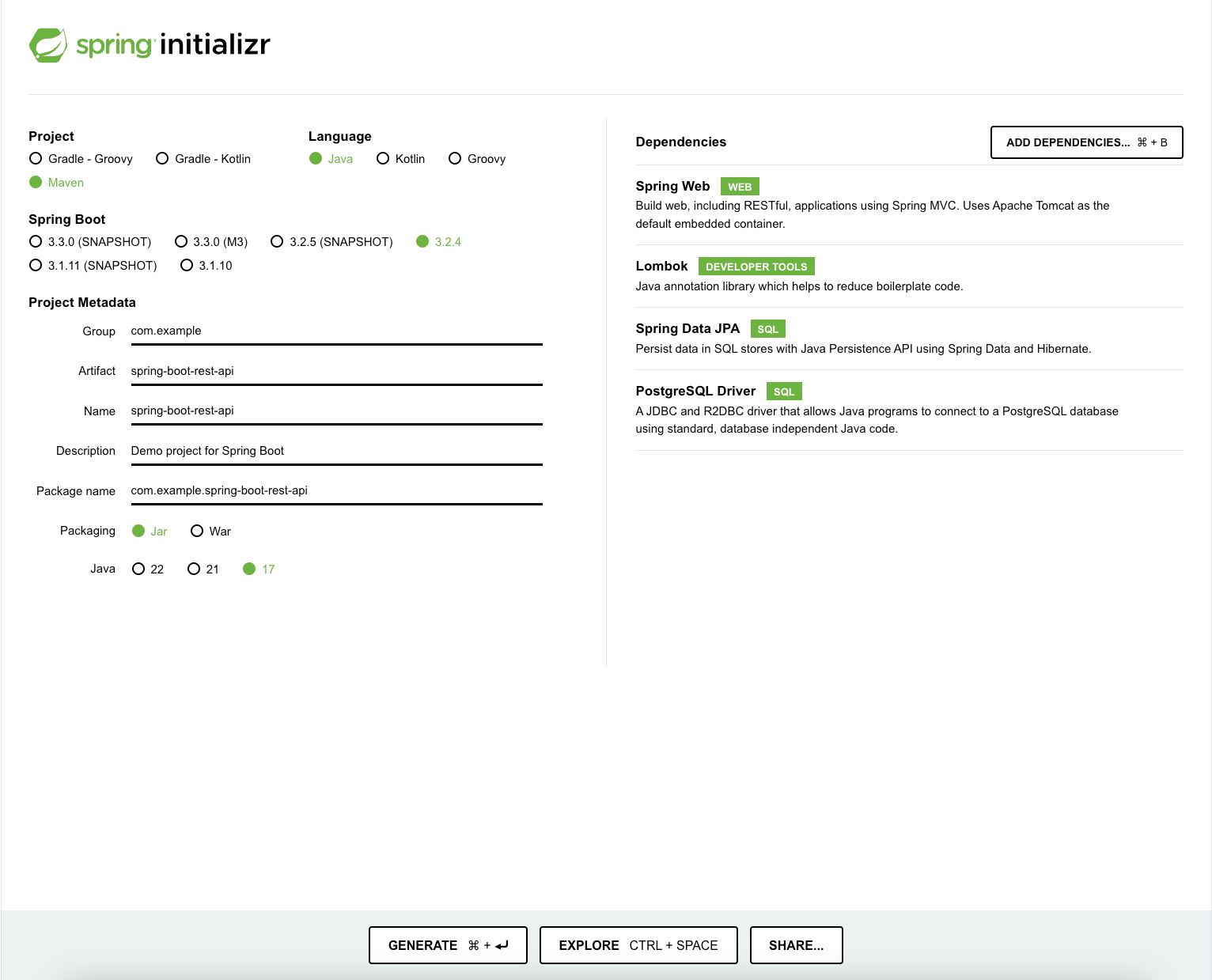
- Opening the downloaded project with your IDE
Alternative, if you are using
Next,
Then, create
IntelliJ Ultimate IDE
, it's easy to create a Spring boot project on your machine 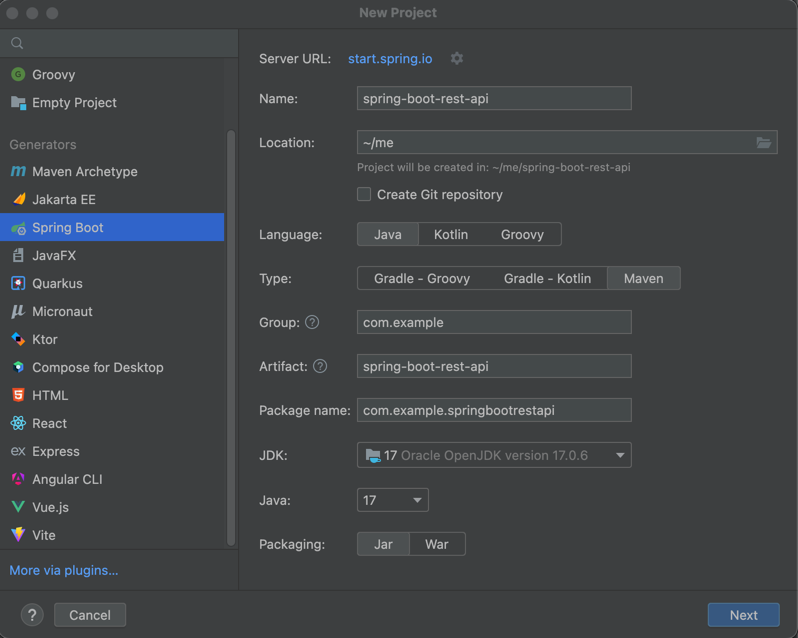
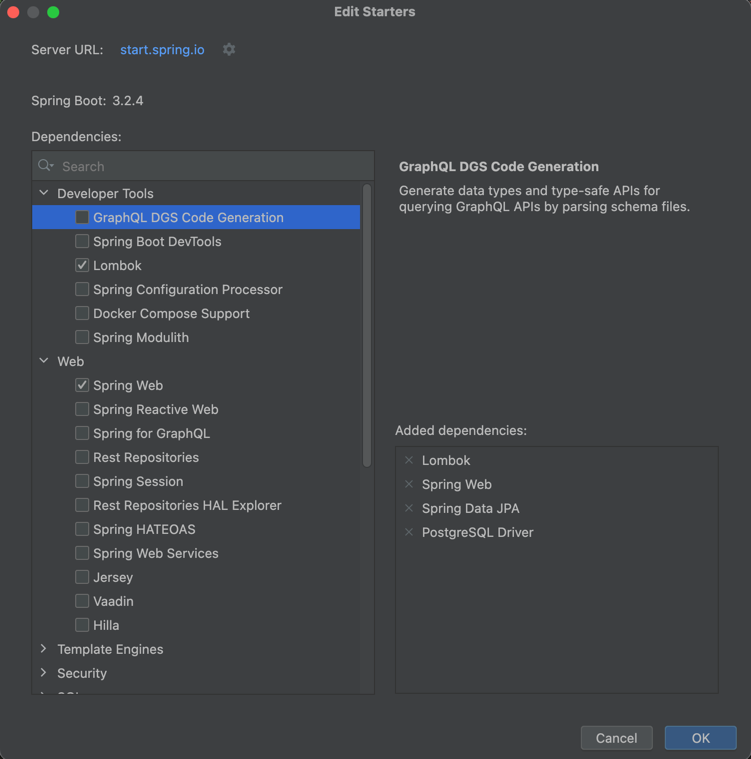
Database setup
Postgresql
can be downloaded via internet and install directly on your machine- Alternatively,
Docker
is also a good option for every developers. Adocker-compose.yml
file is needed and we can easily start and usepostgresql
on your local by commanddocker-compose up
version: '3.5'
name: 'spring-boot-rest-api-docker'
services:
db:
image: postgres:12.13
container_name: postgresql
restart: on-failure
ports:
- 5432:5432
environment:
- POSTGRES_PASSWORD=password
- POSTGRES_USER=postgresql
- POSTGRES_DB=spring-rest-api-db
Okay, then, time to coding ... 👨💻
Architecture
- Controller: a controller is a class responsible for handling incoming HTTP requests and returning an appropriate HTTP response
- Service: a service is a type of class or component that encapsulates business logic or application functionality that can be reused across different parts of an application.
- Repository: a repository is a mechanism or component responsible for handling the storage, retrieval, and management of domain objects or entities.
- Domain: a domain is a representation of domain business objects or entities
Creating a domain object
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
@Entity
@Table(name = "persons")
public class Person {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Integer age;
}
@Getter
: generating the corresponding getter method for the fields@Setter
: generating the corresponding setter method for the fields@NoArgsConstructor
: generating a no-argument constructor for that class@AllArgsConstructor
: generating a all arguments constructor for that class@Entity
: an annotation used in Java Persistence API (JPA) to mark a class as an entity, indicating that instances of this class will be stored in a relational database@Table
: an annotation used in Java Persistence API (JPA) to specify the details of the table to which an entity class will be mapped in the relational database
Creating a controller
PersonController
class is identified by the@RestController
annotationPersonController
class handles aGET
request by@GetMapping
annotation
@RestController
@RequestMapping(PersonController.CONTROLLER_MAPPING)
@RequiredArgsConstructor
public class PersonController {
static final String CONTROLLER_MAPPING = "/person";
private final PersonService personService;
@GetMapping("/list")
public List<Person> getListPerson() {
return personService.getAllPersons();
}
@GetMapping("/{id}")
public Person getById(@PathVariable Long id) {
return personService.getPersonById(id);
}
}
Creating a service
PersonService
is an interface which defines all business methods relevant ofPerson
entity
public interface PersonService {
List<Person> getAllPersons();
Person getPersonById(Long id);
}
PersonServiceImpl
is an implementation, where implements the business logic for all methods. It is identified by@Service
annotation
@Service
@RequiredArgsConstructor
public class PersonServiceImpl implements PersonService {
private final PersonRepository personRepository;
@Override
public List<Person> getAllPersons() {
return personRepository.findAll();
}
@Override
public Person getPersonById(Long id) {
return personRepository.findById(id).orElseThrow(() -> new RuntimeException("Not found"));
}
}
Creating a repository
PersonRepository
is a interface, which extends fromJpaRepository
- an interface provided by the Spring Data JPA framework, which simplifies the implementation of data access layers in Spring applications.
public interface PersonRepository extends JpaRepository<Person, Long> {
Optional<Person> findById(long id);
List<Person> findAll();
}
The final step
- Update your
application.properties
file as below:
spring.application.name=spring-boot-rest-api
spring.datasource.driver-class-name=org.postgresql.Driver
spring.datasource.url=jdbc:postgresql://localhost:5432/spring-rest-api-db
spring.datasource.username=postgresql
spring.datasource.password=password
spring.jpa.database=postgresql
spring.jpa.database-platform=org.hibernate.dialect.PostgreSQLDialect
spring.jpa.hibernate.ddl-auto=create-drop
- Now, this is time for data preparation before see the magic from APIs.
@SpringBootApplication
public class SpringBootRestApiApplication implements CommandLineRunner {
@Autowired
PersonRepository personRepository;
public static void main(String[] args) {
SpringApplication.run(SpringBootRestApiApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
Person person1 = new Person();
person1.setName("John");
person1.setAge(17);
Person person2 = new Person();
person2.setName("Alex");
person2.setAge(18);
List<Person> persons = List.of(person1, person2);
personRepository.saveAll(persons);
}
}
Time to testing ...
Postman is a powerful tool for API testing... Let's download and give it a try
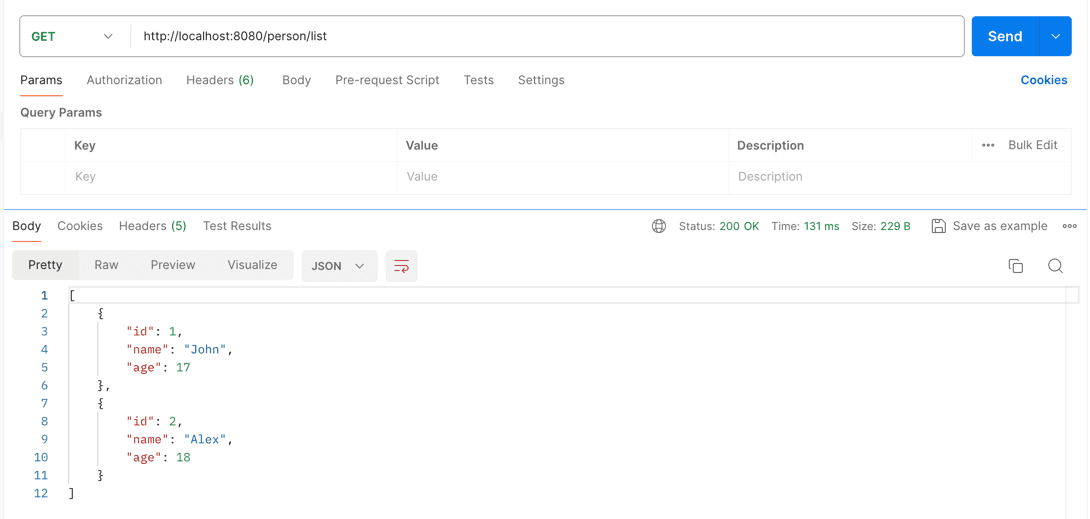
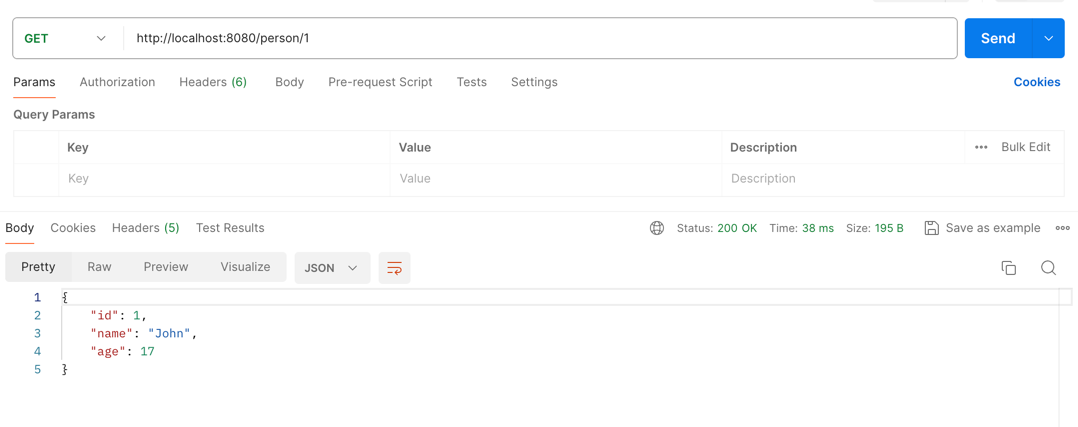